access the object data through webservices
According
to the W3C definition, a "WebService" is "a
software system designed to support interoperable machine-to-machine
interaction over a network. It has an interface described in a machine-processable
format (specifically Web Services Description Language WSDL).
Other systems interact with the Web service in a manner prescribed
by its description using SOAP messages, typically conveyed using
HTTP with an XML serialization in conjunction with other Web-related
standards."
The primary purpose of a webservice is to manipulate
XML representations of Web resources exposing an arbitrary set of
operations.
OBEROn (since version 1.3) employs Apache
CXF to generate the WSDL and to expose/execute the webservice
methods. OBEROn can act both as service provider than service requester.
Service Provider: creating and exposing a Web-Service
Users enabled to manage
WebServices (see user access
rights )
can create a new instance by clicking the "Add new WebService"
button from the "Context Design" menu and compile the
relative form.
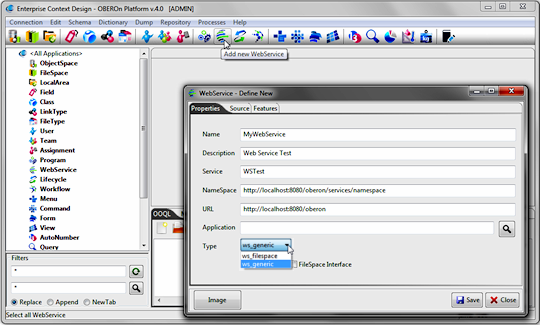
webservice
define 'MyWebService' |
|
description
'Web Service Test'
!hidden !filespace
service 'WSTest'
namespace 'http://localhost:8080/oberon/services/namespace'
url 'http://localhost:8080/oberon' ; |
Name: is the OBEROn internal name (the administration
object name) for the webservice and represents also the generated
java class name.
Description: the webservice description
Service: is the webservice external name; represents the
service name and the WSDL name
NameSpace: is the namespace URL for the webservice; a namespace
is a context for identifiers and contains items which must have
unique names. Items that appear in namespaces have a short (local)
name and unique long "qualified" names for use outside
the name space.
URL: the application server URL where the webservice will
be published; if not specified, the client takes the default parameter
from the oberon.ini configuration file.
Type: shows a list of web-service source templates.
You can add more templates under the .\OBEROn\resources\models folder
; template file names must start with "ws_" and extension
".java" (example: ws_my_weservice_template.java)
A webservice source code has a structure like this:
package com.oberon.webservices;
import javax.xml.ws.WebServiceContext;
import javax.annotation.*;
import javax.jws.*;
@javax.jws.WebService
public class
<CLASSNAME> { |
|
@Resource
private WebServiceContext
context;
@WebMethod
public .... exposeMethod1(@WebParam(name="...")
.... ) { |
|
|
.... CODE .... |
|
} |
|
public ....
Method2(.... ) { |
|
|
.... CODE .... |
|
} |
|
@WebMethod
public .... exposeMethod3(@WebParam(name="...")
.... ) { |
|
|
.... CODE .... |
|
} |
} |
Refer to the "Webservice
Interfaces"
section to know how to write the webservice code.
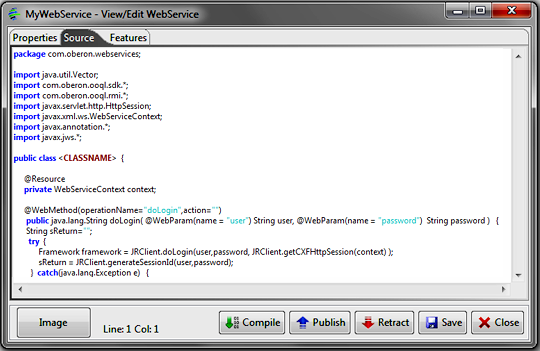
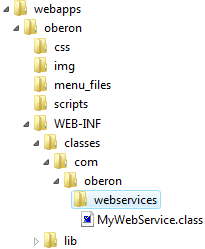
Once the webservice object is created, you can re-open its definition
form and write/compile the code. At the end of this process you
can decide to publish the webservice class into the application
server for a specific OBEROn web-application (referenced by the
URL).
You can do it manually, copying the generated class in the
WEB-INF/classes/com/oberon/webservices folder of the web-application
and edit the WEB-INF/cxf-servlet.xml file for registering
the webservice parameters.
[starting from version 4.3 the name is cxf-oberon.xml]
Otherwise, you can do it in an automatic way, with
the "Publish" button. Pressing this button,
the client compile the source code and calls the JRPublishWS
servlet (it must be registered inside the web-application's configuration
file web.xml) that performs the same operations. [OOQL:
webservice publish 'MyWebService';]
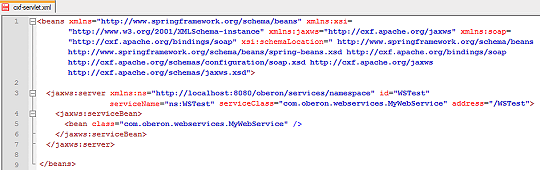
After the web-application re-deploy, you can see the available SOAP
services, including the new web-service at the URL/services address:
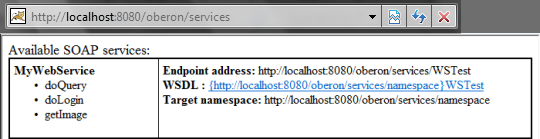
The WSDL describes all the available methods and the
way to access them; the download of the WDSL from the specified
address lets you to create SOAP clients and to perform the web-service's
operations.
If you want to disable the web-service you can manually remove its
registrations from the WEB-INF/cxf-servlet.xml [cxf-oberon.xml] file and (not
necessary) the class from the webservices folder. In a more fast
way you can push the "Retract" button in
the web-service definition form. This calls the JRRetractWS
servlet (it must be registered inside the web-application's configuration
file web.xml) that performs the same operations. [OOQL: webservice
retract 'MyWebService';]
Service Requester: implementing a SOAP client
OBEROn introduces a fast and easy way to create webservice (SOAP)
clients from a service description file (WSDL). The OOQL command:
webservice
clientgen '<WSDL_Address>' ; |
generates all classes which are immediately ready to
be applied into OBEROn programs or triggers.
Example 1:
webservice clientgen "http://localhost:8080/oberon/services/WSTest?wsdl"; |
returns:
import com.oberon.webclients.WSTest.*;
...
MyWebService service = new WSTest().getMyWebServicePort();
class com.oberon.webclients.WSTest.MyWebService
:
|
|
public String doLogin(String
user,String password)
public String doQuery(String sessionId,String classname)
public byte[] getImage(String sessionId,String
classname) |
You can now create e simple program for testing the client:
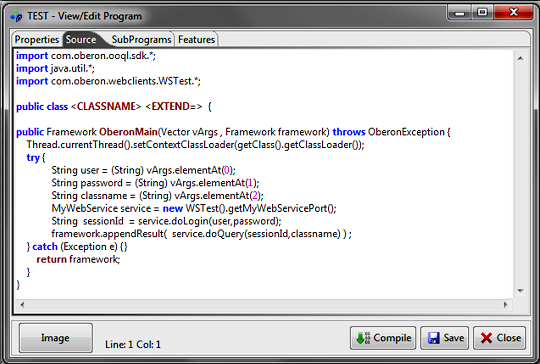
program execute TEST input "test.user"
"test" "SR_Bug";
|
|
SR_Bug|009993455|01|Import Problem - Again|Analysis
SR_Bug|009993455s|00|Import Problem|Test
SR_Bug|43242346|00|Problem Description|Analysis
SR_Bug|02988128|00|Invalid Message|Open
SR_Bug|1234567|00|Error Code #02393|Analysis
SR_Bug|K366545|00|The field is empty|Analysis
SR_Bug|009993455|00|Not closed transaction|Closed
|
You can also employ the generated SOAP client classes
inside the range-programs or trigger-programs to retrieve field-ranges
from another machine over the network or to perform some checks
"on-line".
Example 2:
webservice clientgen "http://wsf.cdyne.com/WeatherWS/Weather.asmx?WSDL"; |
returns:
import com.oberon.webclients.Weather.*;
...
WeatherSoap service = new Weather().getWeatherSoap12();
class com.oberon.webclients.Weather.WeatherSoap: |
|
public com.oberon.webclients.Weather.WeatherReturn getCityWeatherByZIP(String ZIP)
public com.oberon.webclients.Weather.ArrayOfWeatherDescription getWeatherInformation()
public com.oberon.webclients.Weather.ForecastReturn getCityForecastByZIP(String ZIP) |
|
class com.oberon.webclients.Weather.WeatherReturn : |
|
public com.oberon.webclients.Weather.WeatherReturn()
public String getState()
public void setState(String)
public void setDescription(String)
public String getDescription()
public short getWeatherID()
public void setWeatherID(short)
public boolean isSuccess()
public void setSuccess(boolean)
public String getResponseText()
public void setResponseText(String)
public String getCity()
public void setCity(String)
public String getWeatherStationCity()
public void setWeatherStationCity(String)
public String getTemperature()
public void setTemperature(String)
public String getRelativeHumidity()
public void setRelativeHumidity(String)
public String getWind()
public void setWind(String)
public String getPressure()
public void setPressure(String)
public String getVisibility()
public void setVisibility(String)
public String getWindChill()
public void setWindChill(String)
public String getRemarks()
public void setRemarks(String) |
|
class com.oberon.webclients.Weather.ArrayOfWeatherDescription : |
|
public com.oberon.webclients.Weather.ArrayOfWeatherDescription()
public List getWeatherDescription() |
|
class com.oberon.webclients.Weather.ForecastReturn : |
|
public com.oberon.webclients.Weather.ForecastReturn()
public String getState()
public void setState(String)
public boolean isSuccess()
public void setSuccess(boolean)
public String getResponseText()
public void setResponseText(String)
public String getCity()
public void setCity(String)
public String getWeatherStationCity()
public void setWeatherStationCity(String)
public com.oberon.webclients.Weather.ArrayOfForecast getForecastResult()
public void setForecastResult(com.oberon.webclients.Weather.ArrayOfForecast) |
|
class com.oberon.webclients.Weather.ArrayOfForecast : |
|
public com.oberon.webclients.Weather.ArrayOfForecast()
public List getForecast() |
You can now create e simple program for testing the client:
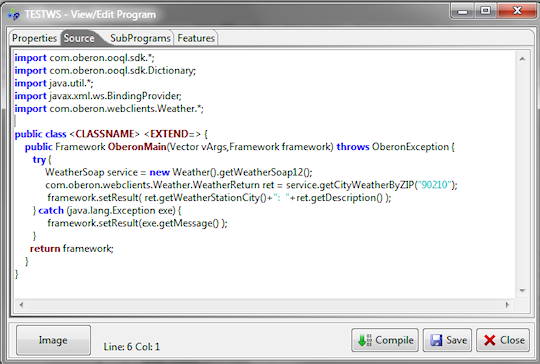
program execute TESTWS ;
|
|
Van Nuys: Sunny
|
The clientgen command supports also the old RCP web-services: in
this case, the client classes are generated by Apache Axis 1 instead
of CXF.
|